Implementing an Android radio button dialog in QPython 3
QPython 3 is a neat app that allows you to run Python 3 scripts on an Android device. All my scripts that I originally created on Linux worked without problems. They were all console scripts though, and I decided to Androidify them a bit. Instead of asking multiple choice questions and making the user type in the answers in the console, the scripts should show nice radio button dialogs:
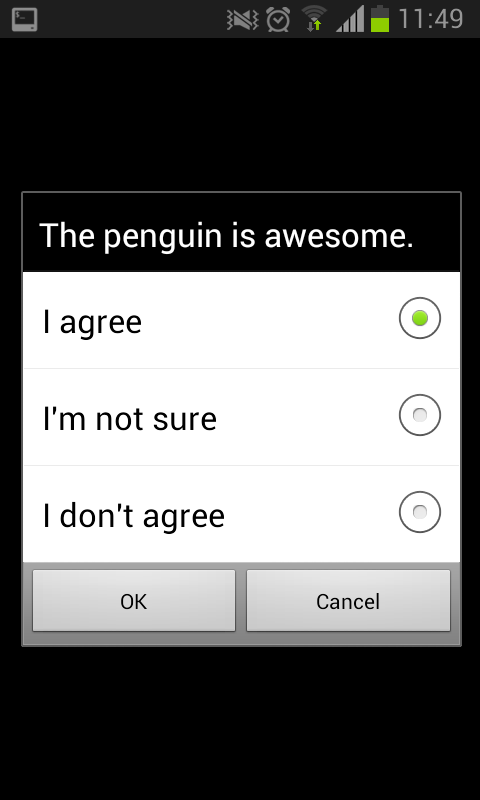
This was not as easy to accomplish as I had hoped. There's not a lot of documentation yet, it's scattered over various websites, and much of it targets Python 2.7. But eventually I got it to work, like this:
import androidhelper
def radioButtonDialog(droid, question, options):
droid.dialogCreateAlert(question)
droid.dialogSetSingleChoiceItems(options)
droid.dialogSetPositiveButtonText("OK")
droid.dialogSetNegativeButtonText("Cancel")
droid.dialogShow()
response = droid.dialogGetResponse().result
if "which" in response:
result = response["which"]
if result == "negative":
raise Exception("Aborted")
selectedItems = droid.dialogGetSelectedItems().result
droid.dialogDismiss()
return selectedItems.pop()
droid = androidhelper.Android()
options = ["I agree", "I'm not sure", "I don't agree"]
try:
result = radioButtonDialog(droid, "The penguin is awesome.", options)
message = "You chose: " + options[result]
except Exception:
message = "Oh, you don't want to tell me. That's ok."
droid.makeToast(message)